Pizza Orders
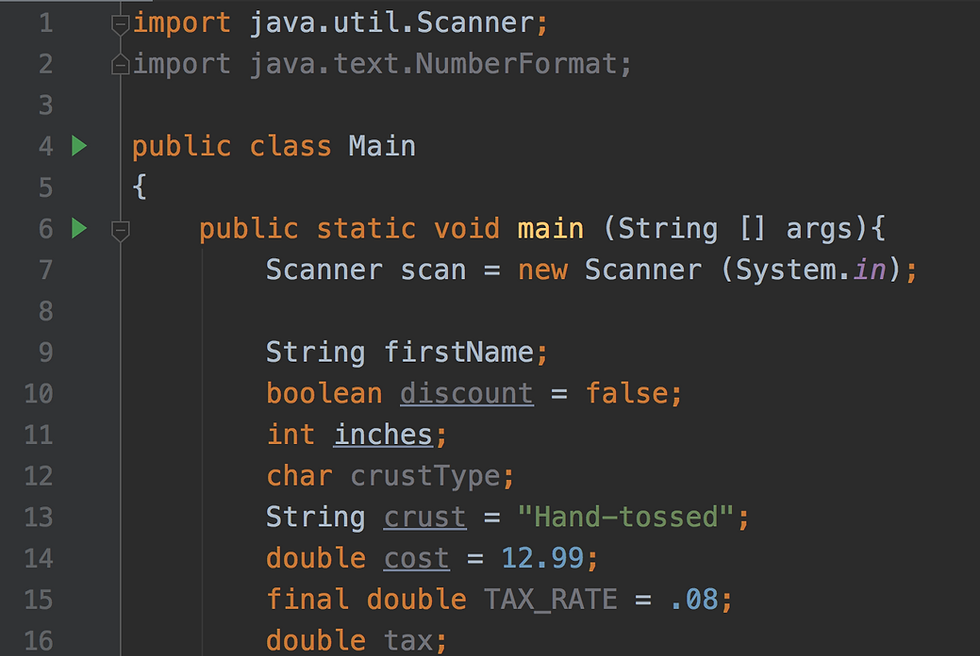
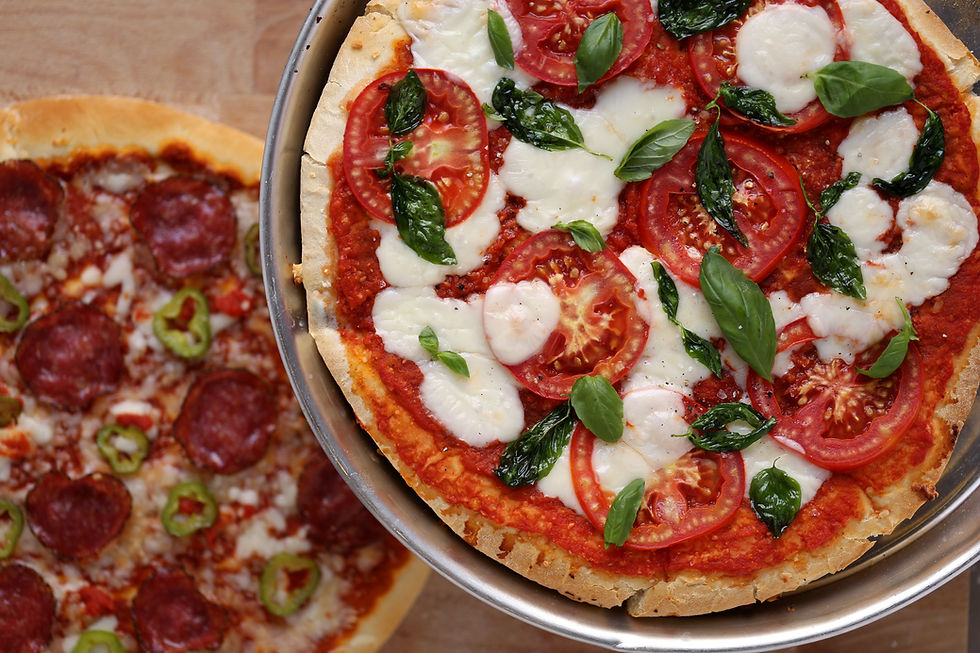
import java.util.Scanner;
import java.text.NumberFormat;
public class Main
{
public static void main (String [] args){
Scanner scan = new Scanner (System.in);
String firstName;
boolean discount = false;
int inches;
char crustType;
String crust = "Hand-tossed";
double cost = 12.99;
final double TAX_RATE = .08;
double tax;
char choice;
String input;
String toppings = "Cheese ";
int numberOfToppings = 0;
System.out.println("Welcome to Rolling Coders Pizza!");
System.out.println("Enter your first name: ");
firstName = scan.nextLine();
if (firstName.equalsIgnoreCase("Megan") || firstName.equalsIgnoreCase("Natalie"))
{
System.out.println("You will receive a discount because your " + "name matches the name of the owner.");
discount = true;
cost /= .15;
}
System.out.println("Pizza size (inches) Cost");
System.out.println(" 10 $10.99");
System.out.println(" 12 $12.99");
System.out.println(" 14 $14.99");
System.out.println(" 16 $16.99");
System.out.println("What size would you like?");
System.out.print("10, 12, 14, 16 (enter the number only):");
inches = scan.nextInt();
if (inches == 10)
{
cost = 10.99;
}
else if (inches == 12)
{
cost = 12.99;
}
else if (inches == 14)
{
cost = 14.99;
}
else if (inches == 16)
{
cost = 16.99;
}
else
{
System.out.println("Please enter a number stated above.");
inches = scan.nextInt();
if (inches == 10)
{
cost = 10.99;
} else if (inches == 12)
{
cost = 12.99;
}
else if (inches == 14)
{
cost = 14.99;
}
else if (inches == 16)
{ cost = 16.99;
}
}
System.out.println("What type of crust do you want?");
System.out.print("(H) Hand-Tossed, (T) Thin-crust, or (D) Deep-Dish (Enter H, T, or D): ");
input = scan.next();
if (input == "H")
{
crust = "Hand-Tossed";
}
else if (input == "T")
{
crust = "Thin-Crust";
}
else if (input == "D")
{
crust = "Deep-Dish";
}
System.out.println("All pizzas come with cheese.");
System.out.println("Additional toppings are $1.25 each, choose from");
System.out.println("(P) Pepperoni, (S) Sausage, (O) Onion, (M) Mushroom");
System.out.print("Do you want pepperoni? (Y/N): ");
input = scan.next();
choice = input.charAt(0);
if (choice == 'Y' || choice == 'y')
{
numberOfToppings += 1;
toppings = toppings + "Pepperoni";
}
}
}