Raspberry Pi Anyone?
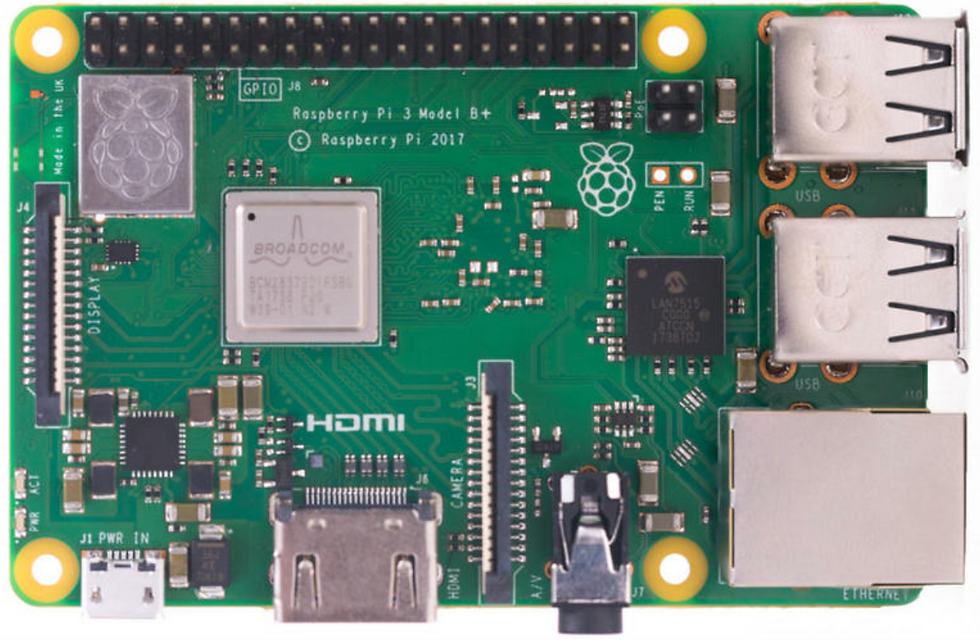
- Let's setup and enable SSH, VNC, etc.
- Customize the desktop
- Update OS:
- sudo apt-get update.sudo
- apt-get dist-upgrade
- Breadboad:
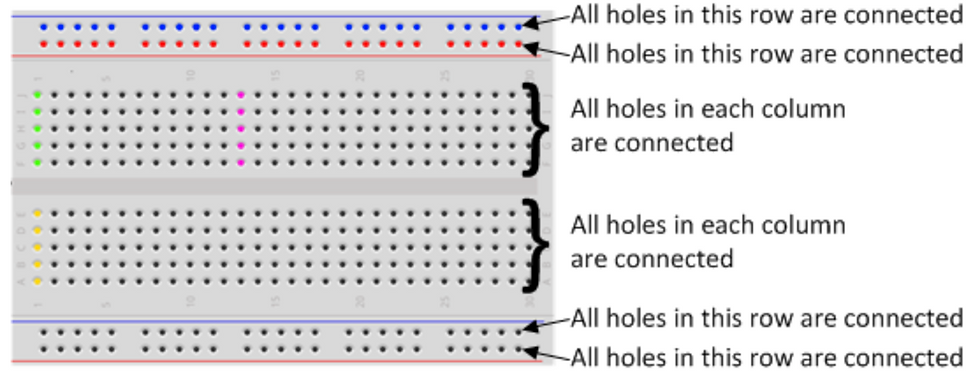
- LED (Light Emitting Diode) [long leg = anode +; short leg = cathode -)
- Resistors are a way of limiting the amount of electricity going through a circuit; specifically, they limit the amount of ‘current’ that is allowed to flow. The measure of resistance is called the Ohm (Ω), and the larger the resistance, the more it limits the current.

- let's blink led:
------
import RPi.GPIO as GPIO import time
GPIO.setmode(GPIO.BCM)
GPIO.setup(17, GPIO.OUT) GPIO.output(17, GPIO.HIGH)
time.sleep(3)
GPIO.output(17, GPIO.LOW) GPIO.cleanup()
---------
import RPi.GPIO as GPIO import time GPIO.setmode(GPIO.BCM) GPIO.setwarnings(False) GPIO.setup(18,GPIO.OUT) print "LED on" GPIO.output(18,GPIO.HIGH) time.sleep(1) print "LED off" GPIO.output(18,GPIO.LOW)
----------
import RPi.GPIO as GPIO # Import Raspberry Pi GPIO library
from time import sleep # Import the sleep function from the time module
GPIO.setwarnings(False) # Ignore warning for now
GPIO.setmode(GPIO.BOARD) # Use physical pin numbering
GPIO.setup(8, GPIO.OUT, initial=GPIO.LOW) # Set pin 8 to be an output pin and set initial value to low (off)
while True: # Run forever
GPIO.output(8, GPIO.HIGH) # Turn on
sleep(1) # Sleep for 1 second
GPIO.output(8, GPIO.LOW) # Turn off
sleep(1) # Sleep for 1 second
------
import RPi.GPIO as GPIO ## Import GPIO library
import time ## Import 'time' library. Allows us to use 'sleep'
GPIO.setmode(GPIO.BOARD) ## Use board pin numbering
GPIO.setup(7, GPIO.OUT) ## Setup GPIO Pin 7 to OUT
##Define a function named Blink()
def Blink(numTimes,speed):
for i in range(0,numTimes):## Run loop numTimes
print ("Iteration " + str(i+1))## Print current loop
GPIO.output(7,True)## Switch on pin 7
time.sleep(speed)## Wait
GPIO.output(7,False)## Switch off pin 7
time.sleep(speed)## Wait
print ("Done") ## When loop is complete, print "Done"
GPIO.cleanup()
## Ask user for total number of blinks and length of each blink
iterations = input("Enter total number of times to blink: ")
speed = input("Enter length of each blink(seconds): ")
## Start Blink() function. Convert user input from strings to numeric data types and pass to Blink() as parameters
Blink(int(iterations),float(speed))
-------