Class and Inheritance
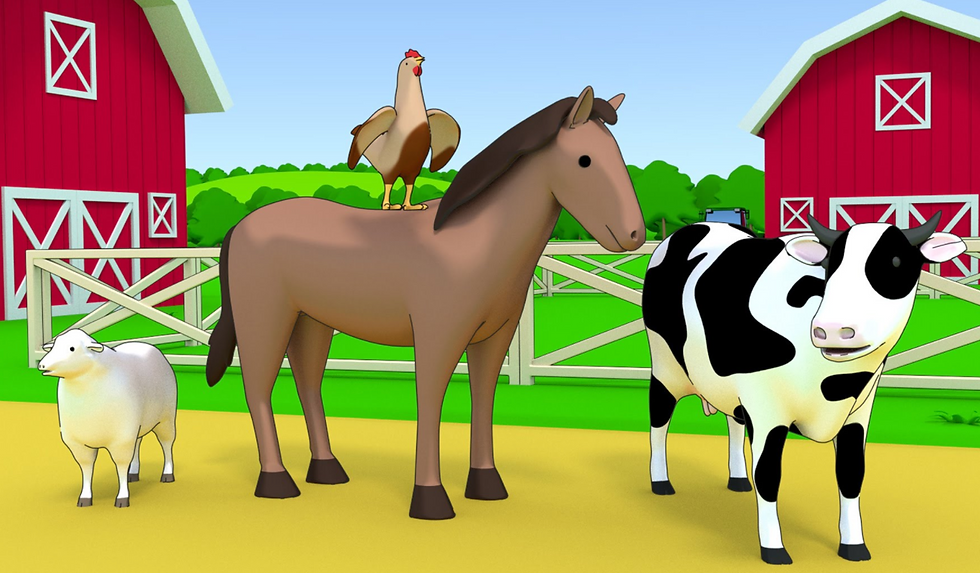
Helper method = method inside the class
- Let's create ordinalAge() to return correct ordinal number - will be called from func message(shouldIncludeAge: Bool)
- How do we get the last digit of a number? For example, 11 is 1, 54 is 4, etc.
- Let's use switch statement to determine if remainder should use st, nd, rd or th.
- Return will be 1st, 2nd, 3rd, 4th, etc.
- Problem: 11, 12, 13 end with 1, but is 11th, 12th, and 13th.
- If not 11, suffix = st
- if not 12, suffix = nd
- if not 13, suffix = rd
- return birthdayAge + Suffix
======
Now, let's make the function "dynamic" to accept Age and Name
====== Inheritance ===
Superclass = Class that other classes inherit from.
Subclass = Class that inherit from Superclass.
Subclass = Can have only 1 Super class.
1. Create class called: FarmAnimal contains String for name = "default farm animal" and Int for numberOfLegs = 4
2. One method called: sayHello() which returns "Hello. I am a farm animal"
3. One method called: description() which returns "I am [name of animal] and I have [#] of legs"
=== Let's Crate a Subclass called sheep ===
1. Create a sub class "Sheep"
2. Override name value of "default farm animal" to "sheep"
3. Override sayHello() to return "baa baa baa"
4. Override description() to return "I provide wool to make blankets."
class FarmAnimal {
var name = "default animal name"
var numberOfLegs = 4
func sayHello() -> String {
return "Hello. I am a farm animal"
}
func description() -> String {
return "I am \(name) and I have \(numberOfLegs) legs."
}
}
class Sheep:FarmAnimal {
override init() {
super.init()
name = "sheep"
}
override func sayHello() -> String {
return "baa baa baa"
}
override func description() -> String {
super.description()
return "I provide wool to make blankets."
}
}
======
1. Use FarmAnimal super class and create sub class chicken
2. Override name = "chicken"
3. Override numberOfLegs = 2
4. Override sayHello method with "bwok bwok bwok"
5. Override description method with "I am a [call variable name] and I have [call variable name] legs."
======
Homework:
- Review class and inheritance.
- Create a super class car with: number of wheels, doors and colors.
- Create the following 3 sub-classes and inherit from the super class: Truck, Car, and Motorcycle
- Prepare and share (3-5 minutes):
1. What do you want to do if you have unlimited resources?
2. How to achieve your goals?
3. What would you be doing in 2018 to move closer to your goals?