My Own Data Type & Code Cake
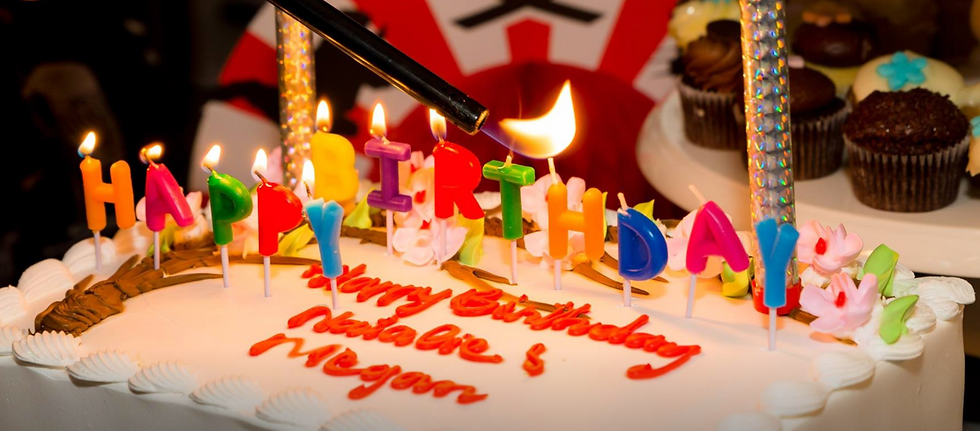
- Class: a package contains data and functions / blueprint creating an object.
- We use class to create objects.
- What are objects?
- State describes the condition of an object.
- A car has color, size, # of seats, etc.
- Behavior describes what an object can do.
- Object created from a class is called an instance.
- Data in a class is the property of the class.
- Function is a class is called class's method.
=== Birthday Cake ==
class BirthdayCake {
let birthdayAge = 10
let bithdayName = "Megan" // This is a default value. Use optional if value not required.
var feeds = 50
}
let myCake = BirthdayCake() // () initializer.
let age = myCake.birthdayAge
myCake.feeds = 100
print("My cake needs \(age) candles")
print("My cake says Happy Birthday to \(myCake.bithdayName)")
print("My cake feeds \(myCake.feeds) people")
- Use dot notation to call the property of the property.
==== Birthday Cake with Initializer NO Parameters =====
class BirthdayCake {
let birthdayAge: Int
let birthdayName: String
var feeds = 20
init() {
birthdayAge = 10
birthdayName = "Megan"
print("Birthday cake for \(birthdayName) is ready")
}
}
==== Birthday Cake with Initializer WITH Parameters =====
class BirthdayCake {
let birthdayAge: Int
let birthdayName: String
var feeds = 20
init(age: Int, name: String) {
birthdayAge = age
birthdayName = name
print("Birthday cake for \(birthdayName) is ready")
}
}
var myCake = BirthdayCake(age: 11, name: "Megan")
==== Birthday Cake Methods ===
class BirthdayCake {
let birthdayAge: Int
let birthdayName: String
var feeds = 20
init(age: Int, name: String) { //value in the class must initialize.
birthdayAge = age
birthdayName = name
// print("Birthday cake for \(birthdayName) is ready.")
}
func message(shouldIncludeAge: Bool) -> String {
if shouldIncludeAge {
return "Happy \(birthdayAge) birthday!"
}
return "Happy Birthday \(birthdayName)"
}
}
var myCake = BirthdayCake(age: 11, name: "Megan")
myCake.message(shouldIncludeAge: false)
var messageInMethod = myCake.message(shouldIncludeAge: false)
print("\(messageInMethod)")
=== Let's write a helper ===
class BirthdayCake {
let birthdayAge: Int
let birthdayName: String
var feeds = 20
init(age: Int, name: String) { //value in the class must initialize.
birthdayAge = age
birthdayName = name
// print("Birthday cake for \(birthdayName) is ready.")
}
func message(shouldIncludeAge: Bool) -> String {
if shouldIncludeAge {
return "Happy \(ordinalAge()) \(birthdayName) birthday!"
}
return "Happy Birthday \(birthdayName)"
}
func ordinalAge() -> String {
var suffix = "th"
let remainderAge = birthdayAge % 10 // find out if the remainder is 1, 2, 3, etc.
switch remainderAge {
case 1:
if birthdayAge != 11 {
suffix = "st"
}
case 2:
if birthdayAge != 12 {
suffix = "nd"
}
case 3:
if birthdayAge != 13 {
suffix = "rd"
}
default:
break
}
return "\(birthdayAge)" + suffix
}
}
var myCake = BirthdayCake(age: 10, name: "Megan")
myCake.message(shouldIncludeAge: false)
var messageInMethod = myCake.message(shouldIncludeAge: true)
print("\(messageInMethod)")
===== Homework ====
- Review class, objects and methods.
- Review all Swift class notes.
- Enjoy 2 weeks off!!!