Let's Scope & Optionals -- Huh?
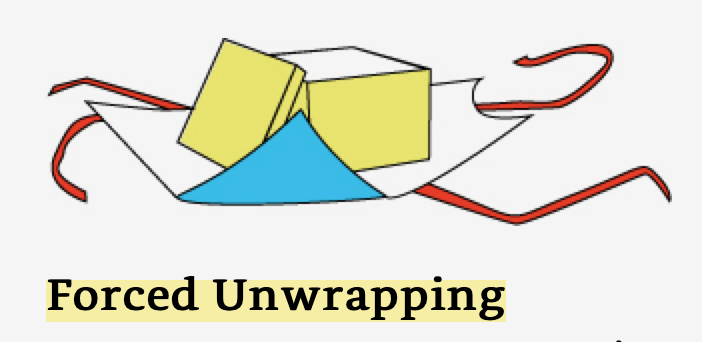
== Scope ==
let isMorning = true
var greeting = ""
if isMorning { greeting = "Good Morning" }
else { greeting = "Good Afternoon" }
print( greeting)
== Optional Value ==
- Think of it as a bag with value or empty (nil)
Example: var teacherName: String?
- Need to unwrap optional value before using it
print("Next year, my teacher is Ms. \(futureTeacher)")
print("Next year, my teacher is Ms. \(futureTeacher!)")
NOTE: force-unwrapping an optional with no value will cause error.
=======
Better way:
var futureTeacher: String?
print(futureTeacher!)
/*if futureTeacher != nil {
let unknownTeacher = futureTeacher!
print("next year my teacher is \(futureTeacher!)")
} else {
print("I don't know who is my teacher")
=====
if-let = to see if optional has a value
**
if let knownTeacher = futureTeacher {
print("Your next year teacher is \(knownTeacher)")
} else {
print("I don't know who is my teacher")
}
==
if let knownTeacher = futureTeacher { print(" Next year \( knownTeacher) will be my teacher.") }
==
if futureTeacher != nil { let knownTeacher = futureTeacher! print(" Next year \( knownTeacher) will be my teacher.")
==
nil coalescing operator ?? HUH ??
When the optional has a value, the value will be used as usual, but when the optional is nil, the nil coalescing operator will use the default value instead.
optionalThing ?? defaultThing
==
let defaultLunch = "Pizza"
var specialLunch: String?
var myLunch = specialLunch ?? defaultLunch
print("On Monday, I had \(myLunch) for lunch")
specialLunch = "Pie"
myLunch = specialLunch ?? defaultLunch
print("On Monday, I had \(myLunch) for lunch")
==
Homework:
- Review this class and the previous classes of Swift
- Make sure you understand the optional variables
- Remember that you need to unwrap the optional variables before using it. But, how?